mirror of
https://github.com/Instadapp/trustwallet-assets.git
synced 2024-07-29 22:37:31 +00:00
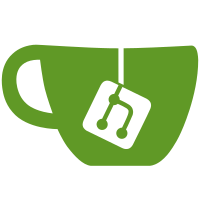
* CMC mapping update. * New check infrastructure, move root folder test to new infra. * Move list of allowed files to config. * Include new check in other tests. * More generic way to call checks. * Organize fix and update actions behind interfaces. * Organize checks into steps, multiple steps per action. * Simplify checkStep class/instance creation. * Migrate chain logo checks. * Migrate asset folder check. * Migrate further chain checks. * Migrate eth fork folder checks. * Migrate binance chain check. * Extra output. * Output improvements. * Async fix. * Migrate Tron check. * Add Tron check. * Remove Tron check from old. * White/blacklist check in new intra, combined with fix. * Refine ETH checks. * Remove from old infra. * Migrate CMC check to new infra. * Migrate validator tests to new check infra. * Migrate Json files validity check to new check infra. * Whitelist check fix. * Cleanup helpers.ts. * Move helpers.ts. * Cleanup of models.ts. * Move models.ts. * Move index.test.ts. * Update with BEP8 support. * Descriptive names for jobs within the builds. Co-authored-by: Catenocrypt <catenocrypt@users.noreply.github.com>
46 lines
1.6 KiB
TypeScript
46 lines
1.6 KiB
TypeScript
import { getChainAssetInfoPath } from "./repo-structure";
|
|
import { readFileSync, isPathExistsSync } from "./filesystem";
|
|
import { arrayDiff } from "./types";
|
|
import { isValidJSON } from "../common/json";
|
|
|
|
const requiredKeys = ["explorer", "name", "website", "short_description"];
|
|
|
|
function isAssetInfoHasAllKeys(path: string): [boolean, string] {
|
|
const info = JSON.parse(readFileSync(path));
|
|
const infoKeys = Object.keys(info);
|
|
|
|
const hasAllKeys = requiredKeys.every(k => info.hasOwnProperty(k));
|
|
|
|
if (!hasAllKeys) {
|
|
return [false, `Info at path '${path}' missing next key(s): ${arrayDiff(requiredKeys, infoKeys)}`];
|
|
}
|
|
|
|
const isKeysCorrentType =
|
|
typeof info.explorer === "string" && info.explorer != ""
|
|
&& typeof info.name === "string" && info.name != ""
|
|
&& typeof info.website === "string"
|
|
&& typeof info.short_description === "string";
|
|
|
|
return [isKeysCorrentType, `Check keys '${requiredKeys}' vs. '${infoKeys}'`];
|
|
}
|
|
|
|
export function isAssetInfoOK(chain: string, address: string): [boolean, string] {
|
|
const assetInfoPath = getChainAssetInfoPath(chain, address);
|
|
if (!isPathExistsSync(assetInfoPath)) {
|
|
return [true, `Info file doesn't exist, no need to check`]
|
|
}
|
|
|
|
if (!isValidJSON(assetInfoPath)) {
|
|
console.log(`JSON at path: '${assetInfoPath}' is invalid`);
|
|
return [false, `JSON at path: '${assetInfoPath}' is invalid`];
|
|
}
|
|
|
|
const [hasAllKeys, msg] = isAssetInfoHasAllKeys(assetInfoPath);
|
|
if (!hasAllKeys) {
|
|
console.log(msg);
|
|
return [false, msg];
|
|
}
|
|
|
|
return [true, ''];
|
|
}
|