mirror of
https://github.com/Instadapp/trustwallet-assets.git
synced 2024-07-29 22:37:31 +00:00
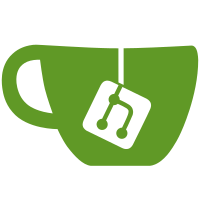
* Empty infrastructure for new-housekeeping build. * Move updateBEP action to new new-housekeeping build infra. * Remove old updateBEP. * New-housekeeping-dryrun run. * Include new top-level folder name script-new. * Remove update:bep2 from old daily-run. * Use imports instead of require. * Small refactor for testability. * Organize scripts into subfolders. * iUpdateBEP2: refactor and add tests. * Move formatting validators to new-housekeeping,add new helpers. * Move info and black/whitelist fixing to new-housekeeping. * New fix command. * New 'fix' target; Move ETH checksum fix to new-housekeeping. * Move logo size check and resize to new-housekeeping. * Improved async error handling. * Build renames. * Move (old) BEP2 and CMC update to periodic update build. * Rename (add missing). * Rename builds. * Renames ('fix'). * rename * Invoke new scripts (as well) from period-update. * Move cmc update to new-periodic. * Move tezos validator update to new-periodic. * Missing file. * Leftover. * Cleanup * Rename of unused openseacontracts. * CMC should not be run always. * Break main/fixAndUpdate function into two. * Show diff in build after changes. * Cleanup * Rename, script-old. * Cleanup, remove old fix build definitions. * Renames, remove new- prefix. * CMC mapping update. * Config infrastructure; add binance URL to config. * Add image size parameters to config. * Rename. Co-authored-by: Catenocrypt <catenocrypt@users.noreply.github.com> Co-authored-by: Andrew M <35627271+zachzwei@users.noreply.github.com>
85 lines
2.5 KiB
TypeScript
85 lines
2.5 KiB
TypeScript
const bluebird = require("bluebird")
|
|
const nestedProperty = require("nested-property");
|
|
import {
|
|
chainsFolderPath,
|
|
getChainInfoPath,
|
|
isChainInfoExistSync,
|
|
writeFileSync,
|
|
readDirSync,
|
|
readFileSync,
|
|
getChainAssetInfoPath,
|
|
getChainAssetsPath,
|
|
isPathExistsSync
|
|
} from "../src/test/helpers"
|
|
import { CoinInfoList } from "../src/test/models";
|
|
|
|
const dafaultInfoTemplate: CoinInfoList =
|
|
{
|
|
"name": "",
|
|
"website": "",
|
|
"source_code": "",
|
|
"whitepaper": "",
|
|
"short_description": "",
|
|
"explorer": "",
|
|
"socials": [
|
|
{
|
|
"name": "Twitter",
|
|
"url": "",
|
|
"handle": ""
|
|
},
|
|
{
|
|
"name": "Reddit",
|
|
"url": "",
|
|
"handle": ""
|
|
}
|
|
],
|
|
"details": [
|
|
{
|
|
"language": "en",
|
|
"description": ""
|
|
}
|
|
]
|
|
}
|
|
|
|
bluebird.mapSeries(readDirSync(chainsFolderPath), (chain: string) => {
|
|
const chainInfoPath = getChainInfoPath(chain)
|
|
|
|
// Create intial info.json file base off template if doesn't exist
|
|
if (!isChainInfoExistSync(chain)) {
|
|
writeToInfo(chainInfoPath, dafaultInfoTemplate)
|
|
}
|
|
|
|
// const infoList: InfoList = JSON.parse(readFileSync(chainInfoPath))
|
|
// delete infoList["data_source"]
|
|
// const newExplorer = nestedProperty.get(infoList, "explorers")
|
|
// console.log({newExplorer}, chain)
|
|
// nestedProperty.set(infoList, "explorer", newExplorer)
|
|
// delete infoList["explorers"]
|
|
// writeToInfo(chainInfoPath, infoList)
|
|
|
|
// if (isPathExistsSync(getChainAssetsPath(chain))) {
|
|
// readDirSync(getChainAssetsPath(chain)).forEach(asset => {
|
|
// const assetInfoPath = getChainAssetInfoPath(chain, asset)
|
|
// if (isPathExistsSync(assetInfoPath)) {
|
|
// const assetInfoList = JSON.parse(readFileSync(assetInfoPath))
|
|
// delete assetInfoList["data_source"]
|
|
|
|
// const newExplorers = nestedProperty.get(assetInfoList, "explorers.0.url")
|
|
// delete assetInfoList["explorers"]
|
|
// nestedProperty.set(assetInfoList, "explorer", newExplorers)
|
|
|
|
// writeToInfo(assetInfoPath, assetInfoList)
|
|
// }
|
|
// })
|
|
// }
|
|
})
|
|
|
|
// Get handle from Twitter and Reddit url
|
|
export function getHandle(url: string): string {
|
|
if (!url) return ""
|
|
return url.slice(url.lastIndexOf("/") + 1, url.length)
|
|
}
|
|
|
|
function writeToInfo(path: string, info: CoinInfoList) {
|
|
writeFileSync(path, JSON.stringify(info, null, 4))
|
|
} |