mirror of
https://github.com/Instadapp/trustwallet-assets.git
synced 2024-07-29 22:37:31 +00:00
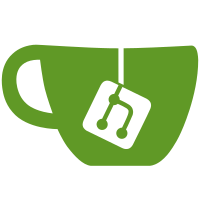
* Add checksum address to tezos validators * Rename tron validators and assets * Optimised images with calibre/image-actions * Uppercase BNB coins * Rename waves * Checksum TT addresses * Checksum POA addresses * Checksum ETC addresses * Checksum Ethreum and POA addresses * Add ethereum-checksum-address * Add BEP2 logos * remove * Fix Ethereum test * Checksum Tomo * Checksum Wanchain * Update ALGO logo * Update test * Update readme * Remove callisto from verify tokens * Update logo.png * Rename tezos * Add web3 * Add new BEP2 images * Uppercase blacklist BEp2 * pdate fetchin opensea nft addreses * test * new * to checksum * Update package * Exclude callisto from test * Checksum the rest * To checksum script * remove * remove * remove * Add * Add jest * Use jest * Breezecoin (BRZE) * Test using Jest * Remove mongoose * Update scritps * 999 * Move dep to dev * Optimised images with calibre/image-actions * Uppercase * To checksum * new * Add babel * Checksum * Move helper meethods to sepaarate file for future reuse * rename vthor * Move js => typescript * Add ts-node * Add default lists * Init blacklist * Fetch opensea assets * Add more helpers * fethc opensea nonerc20 for blacklist * Add list command * script: checksum BEP2 * Rename to ts * Remove old blacklist * Update whitelist and blacklist * Remove tezos validator due to end of service Closes https://github.com/trustwallet/assets/issues/581 * script: generate white and black lists * Add Stakenow to Tezos * Arch Crypton Game (ARCG) * Optimised images with calibre/image-actions * Update list * many updates * Optimised images with calibre/image-actions * Add daps * Optimised images with calibre/image-actions * Update Ethereum blacklist * Utilize trustwallet/types * Remove duplicate * Reflex (RFX) * Remove lowercased
196 lines
6.7 KiB
TypeScript
196 lines
6.7 KiB
TypeScript
const eztz = require('eztz-lib')
|
|
|
|
import {
|
|
Ethereum, Binance, Cosmos, Tezos, Tron, IoTeX, Waves, Classic, POA, TomoChain, GoChain, Wanchain, ThunderCore,
|
|
chainsFolderPath,
|
|
getChainLogoPath,
|
|
getChainAssetsPath,
|
|
getChainAssetLogoPath,
|
|
getChainValidatorsAssets,
|
|
getChainValidatorsListPath,
|
|
getChainValidatorAssetLogoPath,
|
|
readDirSync,
|
|
isPathExistsSync,
|
|
readFileSync,
|
|
isLowerCase,
|
|
isChecksum,
|
|
getBinanceBEP2Symbols,
|
|
isTRC10, isTRC20
|
|
} from "./helpers"
|
|
|
|
describe("Check repository root dir", () => {
|
|
const rootDirAllowedFiles = [
|
|
".github",
|
|
"blockchains",
|
|
"dapps",
|
|
"media",
|
|
"node_modules",
|
|
"script",
|
|
"src",
|
|
".gitignore",
|
|
".travis.yml",
|
|
"jest.config.js",
|
|
"LICENSE",
|
|
"package-lock.json",
|
|
"package.json",
|
|
"README.md",
|
|
".git",
|
|
]
|
|
|
|
const dirActualFiles = readDirSync(".")
|
|
test("Root should contains only predefined files", () => {
|
|
dirActualFiles.forEach(file => {
|
|
expect(rootDirAllowedFiles.indexOf(file), `File "${file}" should not be in root or added to predifined list`).not.toBe(-1)
|
|
})
|
|
})
|
|
})
|
|
|
|
describe(`Test "blockchains" folder`, () => {
|
|
const foundChains = readDirSync(chainsFolderPath)
|
|
|
|
test("Check number of existing chains", () => {
|
|
const supportedChains = 64
|
|
expect(supportedChains).toBe(foundChains.length)
|
|
})
|
|
|
|
test(`Chain should have "logo.png" image`, () => {
|
|
foundChains.forEach(chain => {
|
|
const chainLogoPath = getChainLogoPath(chain)
|
|
expect(isPathExistsSync(chainLogoPath), `File missing at path "${chainLogoPath}"`).toBe(true)
|
|
})
|
|
});
|
|
|
|
test("Chain folder must have lowercase naming", () => {
|
|
foundChains.forEach(chain => {
|
|
expect(isLowerCase(chain), `Chain folder must be in lowercase "${chain}"`).toBe(true)
|
|
})
|
|
});
|
|
|
|
describe("Check Ethereum side-chain folders", () => {
|
|
const ethSidechains = [Ethereum, Classic, POA, TomoChain, GoChain, Wanchain, ThunderCore]
|
|
const ethSidechainSupportedBlacklist = [Ethereum]
|
|
|
|
ethSidechains.forEach(chain => {
|
|
test(`Test chain ${chain} folder`, () => {
|
|
const assetsPath = getChainAssetsPath(chain)
|
|
|
|
readDirSync(assetsPath).forEach(addr => {
|
|
expect(isChecksum(addr), `Address ${addr} on chain ${chain} must be in checksum`).toBe(true)
|
|
|
|
const assetLogoPath = getChainAssetLogoPath(chain, addr)
|
|
expect(isPathExistsSync(assetLogoPath), `Missing file at path "${assetLogoPath}"`).toBe(true)
|
|
})
|
|
})
|
|
})
|
|
})
|
|
|
|
describe(`Check "binace" folder`, () => {
|
|
it("Asset must exist on chain and", async () => {
|
|
const tokenSymbols = await getBinanceBEP2Symbols()
|
|
const assets = readDirSync(getChainAssetsPath(Binance))
|
|
|
|
assets.forEach(asset => {
|
|
expect(tokenSymbols.indexOf(asset), `Asset ${asset} missing on chain`).not.toBe(-1)
|
|
})
|
|
})
|
|
})
|
|
|
|
describe(`Check "tron" folder`, () => {
|
|
const path = getChainAssetsPath(Tron)
|
|
|
|
test("Expect asset to be TRC10 or TRC20", () => {
|
|
readDirSync(path).forEach(asset => {
|
|
expect(isTRC10(asset) || isTRC20(asset), `Asset ${asset} non TRC10 nor TRC20`).toBe(true)
|
|
|
|
const assetsLogoPath = getChainAssetLogoPath(Tron, asset)
|
|
expect(isPathExistsSync(assetsLogoPath), `Missing file at path "${assetsLogoPath}"`).toBe(true)
|
|
});
|
|
})
|
|
})
|
|
|
|
describe("Check Staking chains", () => {
|
|
const stakingChains = [Tezos, Cosmos, IoTeX, Tron, Waves]
|
|
|
|
test("Make sure tests added for new staking chain", () => {
|
|
expect(stakingChains.length).toBe(5)
|
|
})
|
|
|
|
stakingChains.forEach(chain => {
|
|
const validatorsList = JSON.parse(readFileSync(getChainValidatorsListPath(chain)))
|
|
|
|
test(`Make sure ${chain} validators list has correct structure`, () => {
|
|
validatorsList.forEach(val => {
|
|
const keys = Object.keys(val)
|
|
expect(keys.length, `Wrong keys amount`).toBe(4)
|
|
|
|
keys.forEach(key => {
|
|
const type = typeof key
|
|
expect(type, `Wrong key type`).toBe("string")
|
|
})
|
|
})
|
|
})
|
|
|
|
|
|
test(`Chain ${chain} validator must have coresponding asset logo`, () => {
|
|
validatorsList.forEach(({ id }) => {
|
|
const path = getChainValidatorAssetLogoPath(chain, id)
|
|
expect(isPathExistsSync(path), `Chain ${chain} asset ${id} logo must be present at path ${path}`).toBe(true)
|
|
})
|
|
});
|
|
|
|
const chainValidatorsAssetsList = getChainValidatorsAssets(chain)
|
|
switch (chain) {
|
|
case Cosmos:
|
|
testCosmosValidatorsAddress(chainValidatorsAssetsList)
|
|
break;
|
|
case Tezos:
|
|
testTezosValidatorsAssets(chainValidatorsAssetsList)
|
|
break;
|
|
case Tron:
|
|
testTronValidatorsAssets(chainValidatorsAssetsList)
|
|
break;
|
|
case Tron:
|
|
testTronValidatorsAssets(chainValidatorsAssetsList)
|
|
break;
|
|
// TODO Add LOOM
|
|
// TODO Add
|
|
default:
|
|
break;
|
|
}
|
|
|
|
test("Make sure number of validators in the list match validators assets", () => {
|
|
expect(validatorsList.length).toBe(chainValidatorsAssetsList.length)
|
|
});
|
|
})
|
|
|
|
});
|
|
})
|
|
|
|
function testTezosValidatorsAssets(assets) {
|
|
test("Tezos assets must be correctly formated tz1 address", () => {
|
|
assets.forEach(addr => {
|
|
expect(eztz.crypto.checkAddress(addr), `Ivalid Tezos address: ${addr}`).toBe(true)
|
|
});
|
|
})
|
|
}
|
|
|
|
function testTronValidatorsAssets(assets) {
|
|
test("TRON assets must be correctly formated", () => {
|
|
assets.forEach(addr => {
|
|
expect(isTRC20(addr), `Address ${addr} should be TRC20`).toBe(true)
|
|
});
|
|
})
|
|
}
|
|
|
|
function testCosmosValidatorsAddress(assets) {
|
|
test("Cosmos assets must be correctly formated", () => {
|
|
assets.forEach(addr => {
|
|
expect(addr.startsWith("cosmosvaloper1"), `Address ${addr} should start from "cosmosvaloper1"`).toBe(true)
|
|
expect(addr.length, `Address ${addr} should have length 52`).toBe(52)
|
|
expect(isLowerCase(addr), `Address ${addr} should be in lowercase`).toBe(true)
|
|
});
|
|
})
|
|
}
|
|
|
|
// TODO test whitelist
|