mirror of
https://github.com/Instadapp/trustwallet-assets.git
synced 2024-07-29 22:37:31 +00:00
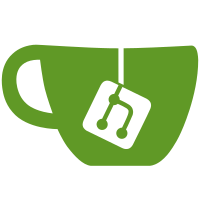
* CMC mapping update. * New check infrastructure, move root folder test to new infra. * Move list of allowed files to config. * Include new check in other tests. * More generic way to call checks. * Organize fix and update actions behind interfaces. * Organize checks into steps, multiple steps per action. * Simplify checkStep class/instance creation. * Migrate chain logo checks. * Migrate asset folder check. * Migrate further chain checks. * Migrate eth fork folder checks. * Migrate binance chain check. * Extra output. * Output improvements. * Async fix. * Migrate Tron check. * Add Tron check. * Remove Tron check from old. * White/blacklist check in new intra, combined with fix. * Refine ETH checks. * Remove from old infra. * Migrate CMC check to new infra. * Migrate validator tests to new check infra. * Migrate Json files validity check to new check infra. * Whitelist check fix. * Cleanup helpers.ts. * Move helpers.ts. * Cleanup of models.ts. * Move models.ts. * Move index.test.ts. * Update with BEP8 support. * Descriptive names for jobs within the builds. Co-authored-by: Catenocrypt <catenocrypt@users.noreply.github.com>
52 lines
3.1 KiB
TypeScript
52 lines
3.1 KiB
TypeScript
import * as path from "path";
|
|
import {
|
|
isPathExistsSync,
|
|
readDirSync
|
|
} from "./filesystem";
|
|
import * as config from "./config";
|
|
|
|
export const logoName = `logo`;
|
|
export const infoName = `info`;
|
|
export const listName = `list`
|
|
export const logoExtension = "png";
|
|
export const jsonExtension = "json";
|
|
export const logoFullName = `${logoName}.${logoExtension}`;
|
|
export const infoFullName = `${infoName}.${jsonExtension}`;
|
|
const whiteList = `whitelist.${jsonExtension}`;
|
|
const blackList = `blacklist.${jsonExtension}`;
|
|
export const validatorsList = `${listName}.${jsonExtension}`
|
|
|
|
export const assetFolderAllowedFiles = [logoFullName, infoFullName];
|
|
export const chainFolderAllowedFiles = [
|
|
"assets",
|
|
whiteList,
|
|
blackList,
|
|
"validators",
|
|
infoName
|
|
]
|
|
export const chainsPath: string = path.join(process.cwd(), '/blockchains');
|
|
export const getChainPath = (chain: string): string => `${chainsPath}/${chain}`;
|
|
export const getChainLogoPath = (chain: string): string => `${getChainPath(chain)}/info/${logoFullName}`;
|
|
export const getChainAssetsPath = (chain: string): string => `${getChainPath(chain)}/assets`;
|
|
export const getChainAssetPath = (chain: string, asset: string) => `${getChainAssetsPath(chain)}/${asset}`;
|
|
export const getChainAssetLogoPath = (chain: string, asset: string): string => `${getChainAssetPath(chain, asset)}/${logoFullName}`;
|
|
export const getChainAssetInfoPath = (chain: string, asset: string): string => `${getChainAssetPath(chain, asset)}/${infoFullName}`;
|
|
export const getChainWhitelistPath = (chain: string): string => `${getChainPath(chain)}/${whiteList}`;
|
|
export const getChainBlacklistPath = (chain: string): string => `${getChainPath(chain)}/${blackList}`;
|
|
export const pricingFolderPath = path.join(process.cwd(), '/pricing');
|
|
|
|
export const getChainValidatorsPath = (chain: string): string => `${getChainPath(chain)}/validators`;
|
|
export const getChainValidatorsListPath = (chain: string): string => `${getChainValidatorsPath(chain)}/${validatorsList}`;
|
|
export const getChainValidatorsAssetsPath = (chain: string): string => `${getChainValidatorsPath(chain)}/assets`
|
|
export const getChainValidatorAssetLogoPath = (chain: string, asset: string): string => `${getChainValidatorsAssetsPath(chain)}/${asset}/${logoFullName}`
|
|
|
|
export const isChainAssetInfoExistSync = (chain: string, address: string) => isPathExistsSync(getChainAssetInfoPath(chain, address));
|
|
|
|
export const getChainFolderFilesList = (chain: string) => readDirSync(getChainPath(chain))
|
|
export const getChainAssetsList = (chain: string): string[] => readDirSync(getChainAssetsPath(chain));
|
|
export const getChainAssetFilesList = (chain: string, address: string) => readDirSync(getChainAssetPath(chain, address));
|
|
export const getChainValidatorsAssets = (chain: string): string[] => readDirSync(getChainValidatorsAssetsPath(chain));
|
|
|
|
const defaultRootDirAllowedFiles = [".github", "blockchains", "dapps", "media", "script", "test", ".gitignore", "LICENSE", "package-lock.json", "package.json", "README.md", ".git", "Gemfile", "Gemfile.lock"];
|
|
export const rootDirAllowedFiles = config.getConfig("folders_rootdir_allowed_files", defaultRootDirAllowedFiles);
|