mirror of
https://github.com/Instadapp/trustwallet-assets.git
synced 2024-07-29 22:37:31 +00:00
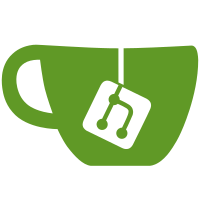
* CMC mapping update. * New check infrastructure, move root folder test to new infra. * Move list of allowed files to config. * Include new check in other tests. * More generic way to call checks. * Organize fix and update actions behind interfaces. * Organize checks into steps, multiple steps per action. * Simplify checkStep class/instance creation. * Migrate chain logo checks. * Migrate asset folder check. * Migrate further chain checks. * Migrate eth fork folder checks. * Migrate binance chain check. * Extra output. * Output improvements. * Async fix. * Migrate Tron check. * Add Tron check. * Remove Tron check from old. * White/blacklist check in new intra, combined with fix. * Refine ETH checks. * Remove from old infra. * Migrate CMC check to new infra. * Migrate validator tests to new check infra. * Migrate Json files validity check to new check infra. * Whitelist check fix. * Cleanup helpers.ts. * Move helpers.ts. * Cleanup of models.ts. * Move models.ts. * Move index.test.ts. * Update with BEP8 support. * Descriptive names for jobs within the builds. Co-authored-by: Catenocrypt <catenocrypt@users.noreply.github.com>
46 lines
1.2 KiB
TypeScript
46 lines
1.2 KiB
TypeScript
import {
|
|
readFileSync,
|
|
writeFileSync
|
|
} from "./filesystem";
|
|
import { sortElements, makeUnique } from "./types";
|
|
|
|
export function isValidJSON(path: string): boolean {
|
|
try {
|
|
let rawdata = readFileSync(path);
|
|
JSON.parse(rawdata);
|
|
return true;
|
|
} catch {
|
|
}
|
|
return false;
|
|
}
|
|
|
|
export function formatJson(content: any) {
|
|
return JSON.stringify(content, null, 4);
|
|
}
|
|
|
|
export function formatSortJson(content: any) {
|
|
return JSON.stringify(sortElements(content), null, 4);
|
|
}
|
|
|
|
export function formatUniqueSortJson(content: any) {
|
|
return JSON.stringify(makeUnique(sortElements(content)), null, 4);
|
|
}
|
|
|
|
export function formatJsonFile(filename: string, silent: boolean = false) {
|
|
writeFileSync(filename, formatJson(JSON.parse(readFileSync(filename))));
|
|
console.log(`Formatted json file ${filename}`);
|
|
}
|
|
|
|
export function formatSortJsonFile(filename: string) {
|
|
writeFileSync(filename, formatSortJson(JSON.parse(readFileSync(filename))));
|
|
console.log(`Formatted json file ${filename}`);
|
|
}
|
|
|
|
export function readJsonFile(path: string): any {
|
|
return JSON.parse(readFileSync(path));
|
|
}
|
|
|
|
export function writeJsonFile(path: string, data: any) {
|
|
writeFileSync(path, JSON.stringify(data, null, 4));
|
|
}
|