mirror of
https://github.com/Instadapp/trustwallet-assets.git
synced 2024-07-29 22:37:31 +00:00
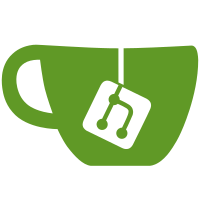
* CMC mapping update. * New check infrastructure, move root folder test to new infra. * Move list of allowed files to config. * Include new check in other tests. * More generic way to call checks. * Organize fix and update actions behind interfaces. * Organize checks into steps, multiple steps per action. * Simplify checkStep class/instance creation. * Migrate chain logo checks. * Migrate asset folder check. * Migrate further chain checks. * Migrate eth fork folder checks. * Migrate binance chain check. * Extra output. * Output improvements. * Async fix. * Migrate Tron check. * Add Tron check. * Remove Tron check from old. * White/blacklist check in new intra, combined with fix. * Refine ETH checks. * Remove from old infra. * Migrate CMC check to new infra. * Migrate validator tests to new check infra. * Migrate Json files validity check to new check infra. * Whitelist check fix. * Cleanup helpers.ts. * Move helpers.ts. * Cleanup of models.ts. * Move models.ts. * Move index.test.ts. * Update with BEP8 support. * Descriptive names for jobs within the builds. Co-authored-by: Catenocrypt <catenocrypt@users.noreply.github.com>
44 lines
1.8 KiB
TypeScript
44 lines
1.8 KiB
TypeScript
import * as fs from "fs";
|
|
import * as path from "path";
|
|
import { execSync } from "child_process";
|
|
|
|
export const getFileName = (name: string): string => path.basename(name, path.extname(name))
|
|
export const getFileExt = (name: string): string => name.slice((Math.max(0, name.lastIndexOf(".")) || Infinity) + 1)
|
|
|
|
export const readFileSync = (path: string) => fs.readFileSync(path, 'utf8');
|
|
export const writeFileSync = (path: string, data: any) => fs.writeFileSync(path, data);
|
|
export const readDirSync = (path: string): string[] => fs.readdirSync(path);
|
|
export const isPathExistsSync = (path: string): boolean => fs.existsSync(path);
|
|
export const getFileSizeInKilobyte = (path: string): number => fs.statSync(path).size / 1000;
|
|
|
|
function execRename(command: string, cwd: string) {
|
|
console.log(`Running command ${command}`);
|
|
execSync(command, {encoding: "utf-8", cwd: cwd});
|
|
}
|
|
|
|
function gitMoveCommand(oldName: string, newName: string): string {
|
|
return `git mv ${oldName} ${newName}-temp && git mv ${newName}-temp ${newName}`;
|
|
}
|
|
|
|
export function gitMove(path: string, oldName: string, newName: string) {
|
|
console.log(`Renaming file or folder at path ${path}: ${oldName} => ${newName}`);
|
|
execRename(gitMoveCommand(oldName, newName), path);
|
|
}
|
|
|
|
export function findFiles(base: string, ext: string, files: string[] = [], result: string[] = []): string[] {
|
|
files = fs.readdirSync(base) || files;
|
|
result = result || result;
|
|
|
|
files.forEach(file => {
|
|
var newbase = path.join(base, file);
|
|
if (fs.statSync(newbase).isDirectory()) {
|
|
result = findFiles(newbase, ext, fs.readdirSync(newbase), result);
|
|
} else {
|
|
if (file.substr(-1*(ext.length+1)) == '.' + ext) {
|
|
result.push(newbase);
|
|
}
|
|
}
|
|
});
|
|
return result;
|
|
}
|