mirror of
https://github.com/Instadapp/trustwallet-assets.git
synced 2024-07-29 22:37:31 +00:00
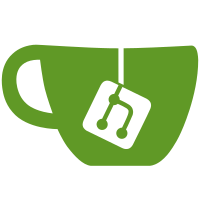
* Make non-mandatory action interface elements optional. * Remove one unused import * Scripts main renamed to entrypoint. * Script common rename to generic * Move generic scripts from action to generic. * Move chain-specific scripts to blockchain. Co-authored-by: Catenocrypt <catenocrypt@users.noreply.github.com>
46 lines
1.6 KiB
TypeScript
46 lines
1.6 KiB
TypeScript
import { getChainAssetInfoPath } from "./repo-structure";
|
|
import { readFileSync, isPathExistsSync } from "./filesystem";
|
|
import { arrayDiff } from "./types";
|
|
import { isValidJSON } from "../generic/json";
|
|
|
|
const requiredKeys = ["explorer", "name", "website", "short_description"];
|
|
|
|
function isAssetInfoHasAllKeys(path: string): [boolean, string] {
|
|
const info = JSON.parse(readFileSync(path));
|
|
const infoKeys = Object.keys(info);
|
|
|
|
const hasAllKeys = requiredKeys.every(k => Object.prototype.hasOwnProperty.call(info, k));
|
|
|
|
if (!hasAllKeys) {
|
|
return [false, `Info at path '${path}' missing next key(s): ${arrayDiff(requiredKeys, infoKeys)}`];
|
|
}
|
|
|
|
const isKeysCorrentType =
|
|
typeof info.explorer === "string" && info.explorer != ""
|
|
&& typeof info.name === "string" && info.name != ""
|
|
&& typeof info.website === "string"
|
|
&& typeof info.short_description === "string";
|
|
|
|
return [isKeysCorrentType, `Check keys '${requiredKeys}' vs. '${infoKeys}'`];
|
|
}
|
|
|
|
export function isAssetInfoOK(chain: string, address: string): [boolean, string] {
|
|
const assetInfoPath = getChainAssetInfoPath(chain, address);
|
|
if (!isPathExistsSync(assetInfoPath)) {
|
|
return [true, `Info file doesn't exist, no need to check`]
|
|
}
|
|
|
|
if (!isValidJSON(assetInfoPath)) {
|
|
console.log(`JSON at path: '${assetInfoPath}' is invalid`);
|
|
return [false, `JSON at path: '${assetInfoPath}' is invalid`];
|
|
}
|
|
|
|
const [hasAllKeys, msg] = isAssetInfoHasAllKeys(assetInfoPath);
|
|
if (!hasAllKeys) {
|
|
console.log(msg);
|
|
return [false, msg];
|
|
}
|
|
|
|
return [true, ''];
|
|
}
|