mirror of
https://github.com/Instadapp/trustwallet-assets.git
synced 2024-07-29 22:37:31 +00:00
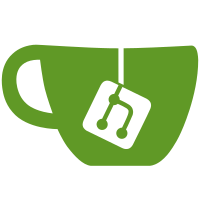
* Add checksum address to tezos validators * Rename tron validators and assets * Optimised images with calibre/image-actions * Uppercase BNB coins * Rename waves * Checksum TT addresses * Checksum POA addresses * Checksum ETC addresses * Checksum Ethreum and POA addresses * Add ethereum-checksum-address * Add BEP2 logos * remove * Fix Ethereum test * Checksum Tomo * Checksum Wanchain * Update ALGO logo * Update test * Update readme * Remove callisto from verify tokens * Update logo.png * Rename tezos * Add web3 * Add new BEP2 images * Uppercase blacklist BEp2 * pdate fetchin opensea nft addreses * test * new * to checksum * Update package * Exclude callisto from test * Checksum the rest * To checksum script * remove * remove * remove * Add * Add jest * Use jest * Breezecoin (BRZE) * Test using Jest * Remove mongoose * Update scritps * 999 * Move dep to dev * Optimised images with calibre/image-actions * Uppercase * To checksum * new * Add babel * Checksum * Move helper meethods to sepaarate file for future reuse * rename vthor * Move js => typescript * Add ts-node * Add default lists * Init blacklist * Fetch opensea assets * Add more helpers * fethc opensea nonerc20 for blacklist * Add list command * script: checksum BEP2 * Rename to ts * Remove old blacklist * Update whitelist and blacklist * Remove tezos validator due to end of service Closes https://github.com/trustwallet/assets/issues/581 * script: generate white and black lists * Add Stakenow to Tezos * Arch Crypton Game (ARCG) * Optimised images with calibre/image-actions * Update list * many updates * Optimised images with calibre/image-actions * Add daps * Optimised images with calibre/image-actions * Update Ethereum blacklist * Utilize trustwallet/types * Remove duplicate * Reflex (RFX) * Remove lowercased
73 lines
3.4 KiB
TypeScript
73 lines
3.4 KiB
TypeScript
import * as fs from "fs"
|
|
const axios = require('axios')
|
|
const Web3 = require('web3')
|
|
const web3 = new Web3('ws://localhost:8546');
|
|
import { CoinTypeUtils, CoinType } from "@trustwallet/types";
|
|
|
|
export const Binance = getChainName(CoinType.binance)
|
|
export const Cosmos = getChainName(CoinType.cosmos)
|
|
export const Ethereum = getChainName(CoinType.ethereum)
|
|
export const Tezos = getChainName(CoinType.tezos)
|
|
export const Tron = getChainName(CoinType.tron)
|
|
export const IoTeX = getChainName(CoinType.iotex)
|
|
export const Waves = getChainName(CoinType.waves)
|
|
export const Classic = getChainName(CoinType.classic)
|
|
export const POA = getChainName(CoinType.poa)
|
|
export const TomoChain = getChainName(CoinType.tomochain)
|
|
export const GoChain = getChainName(CoinType.gochain)
|
|
export const Wanchain = getChainName(CoinType.wanchain)
|
|
export const ThunderCore = getChainName(CoinType.thundertoken)
|
|
|
|
const whiteList = 'whitelist.json'
|
|
const blackList = 'blacklist.json'
|
|
|
|
export const logo = `logo.png`
|
|
export const chainsFolderPath = './blockchains'
|
|
export const getChainLogoPath = chain => `${chainsFolderPath}/${chain}/info/${logo}`
|
|
export function getChainAssetsPath (chain) {
|
|
return `${chainsFolderPath}/${chain}/assets`
|
|
}
|
|
|
|
export const getChainAssetLogoPath = (chain, address) => `${getChainAssetsPath(chain)}/${address}/${logo}`
|
|
export const getChainValidatorsPath = chain => `${chainsFolderPath}/${chain}/validators`
|
|
export const getChainValidatorsAssets = chain => readDirSync(getChainValidatorsAssetsPath(chain))
|
|
export const getChainValidatorsListPath = chain => `${(getChainValidatorsPath(chain))}/list.json`
|
|
export const getChainValidatorsAssetsPath = chain => `${getChainValidatorsPath(chain)}/assets`
|
|
export const getChainValidatorAssetLogoPath = (chain, asset) => `${getChainValidatorsAssetsPath(chain)}/${asset}/${logo}`
|
|
export const getChainWhitelistPath = chain => `${chainsFolderPath}/${chain}/${whiteList}`
|
|
export const getChainBlacklistPath = chain => `${chainsFolderPath}/${chain}/${blackList}`
|
|
|
|
export const readDirSync = path => fs.readdirSync(path)
|
|
export const isPathExistsSync = path => fs.existsSync(path)
|
|
export const isChainWhitelistExistSync = chain => isPathExistsSync(getChainWhitelistPath(chain))
|
|
export const isChainBlacklistExistSync = chain => isPathExistsSync(getChainBlacklistPath(chain))
|
|
export const readFileSync = path => fs.readFileSync(path, 'utf8')
|
|
export const writeFileSync = (path, str) => fs.writeFileSync(path, str)
|
|
|
|
export const isLowerCase = str => str.toLowerCase() === str
|
|
export const isUpperCase = str => str.toUpperCase() === str
|
|
export const isChecksum = address => web3.utils.checkAddressChecksum(address)
|
|
export const toChecksum = address => web3.utils.toChecksumAddress(address)
|
|
export const getBinanceBEP2Symbols = async () => axios.get(`https://dex-atlantic.binance.org/api/v1/tokens?limit=1000`).then(res => res.data.map(({symbol}) => symbol))
|
|
|
|
export const isTRC10 = id => (/^\d+$/.test(id))
|
|
export const isTRC20 = address => {
|
|
return address.length == 34 &&
|
|
address.startsWith("T") &&
|
|
isLowerCase(address) == false &&
|
|
isUpperCase(address) == false
|
|
}
|
|
|
|
export const sortDesc = arr => arr.sort((a, b) => a - b)
|
|
export const getUnique = arr => Array.from(new Set(arr))
|
|
export const mapList = arr => {
|
|
return arr.reduce((acm, val) => {
|
|
acm[val] = ""
|
|
return acm
|
|
}, {})
|
|
}
|
|
|
|
function getChainName(id: CoinType): string {
|
|
return CoinTypeUtils.id(id)
|
|
}
|